poolcontroller
¶
This module is part of the Python Pool library. It defines the base classes for
Classes
PoolController¶
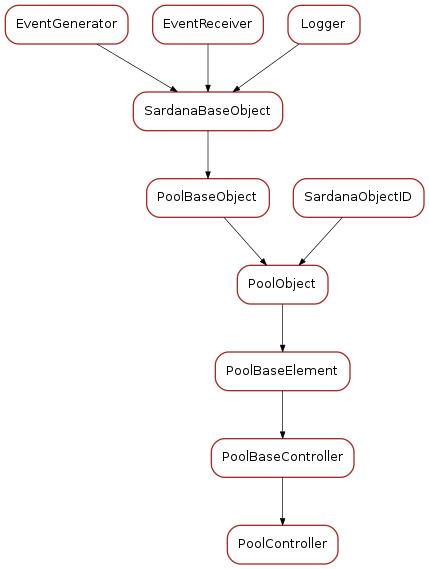
-
class
PoolController
(**kwargs)[source]¶ Bases:
sardana.pool.poolcontroller.PoolBaseController
Controller class mediator for sardana controller plugins
-
ctrl
¶ actual controller object
-
ctrl_info
¶ controller information object
-
set_operator
(operator)[source]¶ Defines the current operator object for this controller. For example, in acquisition, it should be a
PoolMeasurementGroup
object.Parameters: operator (object) – the new operator object
-
operator
¶ current controller operator
-
raw_read_axis_states
(axes=None, ctrl_states=None)[source]¶ Unsafe method. Reads the state for the given axes. If axes is None, reads the state of all active axes.
Parameters: axes (seq<int> or None) – the list of axis to get the state. Default is None meaning all active axis in this controller Returns: a tuple of two elements: a map containing the controller state information for each axis and a boolean telling if an error occured Return type: dict<PoolElement, state info>, bool
-
read_axis_states
(*args, **kwargs)[source]¶ Reads the state for the given axes. If axes is None, reads the state of all active axes.
Parameters: axes (seq<int> or None) – the list of axis to get the state. Default is None meaning all active axis in this controller Returns: a map containing the controller state information for each axis Return type: dict<PoolElement, state info>
-
raw_read_axis_values
(axes=None, ctrl_values=None)[source]¶ Unsafe method. Reads the value for the given axes. If axes is None, reads the value of all active axes.
Parameters: axes (seq<int> or None) – the list of axis to get the value. Default is None meaning all active axis in this controller Returns: a map containing the controller value information for each axis Return type: dict<PoolElement, SardanaValue>
-
read_axis_values
(*args, **kwargs)[source]¶ Reads the value for the given axes. If axes is None, reads the value of all active axes.
Parameters: axes (seq<int> or None) – the list of axis to get the value. Default is None meaning all active axis in this controller Returns: a map containing the controller value information for each axis Return type: dict<PoolElement, SardanaValue>
-
stop_axes
(axes)[source]¶ Stops the given axes.
Parameters: axes (list<axes>) – the list of axes to stopped. Returns: list of axes that could not be stopped Return type: list<int>
-
stop_element
(*args, **kwargs)[source]¶ Stops the given element.
Parameters: element (PoolElement) – the list of elements to stop Raises: Exception – not able to stop element
-
stop_elements
(*args, **kwargs)[source]¶ Stops the given elements. If elements is None, stops all active elements.
Parameters: elements (seq<PoolElement> or None) – the list of elements to stop. Default is None meaning all active elements in this controller Returns: list of elements that could not be stopped Return type: list<PoolElements>
-
stop
(*args, **kwargs)¶ Stops the given elements. If elements is None, stops all active elements.
Parameters: elements (seq<PoolElement> or None) – the list of elements to stop. Default is None meaning all active elements in this controller Returns: list of elements that could not be stopped Return type: list<PoolElements>
-
abort_axes
(*args, **kwargs)[source]¶ Aborts the given axes.
Parameters: axes (list<axes>) – the list of axes to aborted. Returns: list of axes that could not be aborted Return type: list<int>
-
abort_element
(*args, **kwargs)[source]¶ Aborts the given elements.
Parameters: element (PoolElement) – the list of elements to abort Raises: Exception – not able to abort element
-
abort_elements
(*args, **kwargs)[source]¶ Abort the given elements. If elements is None, stops all active elements.
Parameters: elements (seq<PoolElement> or None) – the list of elements to stop. Default is None meaning all active elements in this controller Returns: list of elements that could not be aborted Return type: list<PoolElements>
-
abort
(*args, **kwargs)¶ Abort the given elements. If elements is None, stops all active elements.
Parameters: elements (seq<PoolElement> or None) – the list of elements to stop. Default is None meaning all active elements in this controller Returns: list of elements that could not be aborted Return type: list<PoolElements>
-
emergency_break
(*args, **kwargs)[source]¶ Stops the given elements. If elements is None, stops all active elements. If stop could not be executed, an abort is attempted.
Parameters: elements – the list of elements to stop. Default is None meaning all active elements in this controller Returns: elements that could neither be stopped nor aborted Return type: list<PoolElement>
-